Der bekannte Hamming-Code ist eine Testtechnik, die die Datenintegrität überprüft, wenn Informationspakete von einem Sendergerät zu einem Empfängergerät transportiert werden. Ich habe ein Programm in der Dev-C ++ – CPP-Umgebung geschrieben, das Datenzeichenfolgen im folgenden Format X1.X2.X3.X4 verwendet und das gesamte Array in den entsprechenden Hamming-Codestandard konvertiert. Unsere Testmethode basiert auf der Paritätsprüfung und liefert Ergebnisse in Form von Bit-Arrays (0 und 1) und mathematischen Ausdrücken (Polynomgleichungen). Insgesamt kann der entwickelte Algorithmus Daten bis zu 128 Bit verarbeiten.
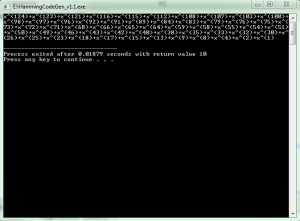
Mein Hamming Code Generator zur Redundanzprüfung kann von hier runtergeladen werden.
/*
* Name: Hamming code generator code for redundancy checking
* Author: Sorin Liviu Jurj
*
* Description:
*
*
*
*
*
*
*/
#include<stdio.h>
#include<math.h>
int isPowerOfTwo(int n) ;
/*array to which hamming code is generated*/
char hchar_a[25];
/**
* FUNCTION NAME : main
*
* FILENAME : HammingCodeGen_v1.1.c
*
* @param : void
*
* @brief : main startup fun
*
* @return : void
*/
void main(){
int r,RedBits,i = 0 , j = 0;
char bit;
char setFlag = 0;
/*the array for which the hamming code has to be generated*/
// char mChar[] = {0x90,0x11};
// int len = 2; //Length of the array
char mChar[] = „421.417.395.423“;
int len = 15; //Length of the array
int curbitNo = 0,tmpcurbitNo ; //store bitposition of the array
int offset = 0,tmpoffset; //store offset of the array
int bitnum = 1;
int totalbits = 0 ;
char temp = 0;
/*find the number of redundant bits and total num of bits req for hamming code */
len = len *8;
for(RedBits = 0 ; RedBits < len ; RedBits ++){
if((pow(2,RedBits) – RedBits) >= len + 1)
break;
}
//printf(„%d \n“,RedBits);
totalbits = len + RedBits;
/*save only data bits into the output array skipping the positions where redundant bits has to be saved*/
/*offset gives the index of output array and curbitNo is the bit position of that array*/
for(j=0 ; j < len / 8 ; j++){
for( i = 7 ; i >= 0 ; i–){
bit = ( mChar[j] >> i ) & 1;
L1:
if(curbitNo == 8 ){
curbitNo = 0 ;
offset++;
hchar_a[offset] = 0x00;
}
if(isPowerOfTwo(totalbits-bitnum+1 )){
bitnum++;
curbitNo++;
goto L1;
}
//printf(„bit %x ,totalbits-bitnum : %d \n“,bit,totalbits-bitnum+1);
hchar_a[offset] = hchar_a[offset] | (bit << (7-curbitNo));
hchar_a[offset]&= 0x000000ff;
bitnum++;
curbitNo++;
//printf(„no red hChar %x\n“,hchar_a[offset]);
}
}
//printf(„offf : %d\n „,offset);
/*
* find the redundant bit position and parity using the logic specified in the link below
* ref : https://www.geeksforgeeks.org/computer-network-hamming-code/
* even parity is used here
*/
for(r = 0 ; r < RedBits ; r++){
offset = 0;
curbitNo = 0;
for(i=totalbits; i>=1 ; i–){
if(i == pow(2,r)){
tmpcurbitNo = curbitNo;
tmpoffset = offset;
}
if( !isPowerOfTwo(i) && (i&(1<<r))){
temp = (hchar_a[offset]) >> (7-curbitNo) & 1 ? temp+1 : temp;
//printf(„i %x, val : %x\n“,i,(hChar >> i-1) & 1);
}
curbitNo++;
if(curbitNo == 8){
curbitNo = 0;
offset++;
}
}
/*find the parity by checking the number of one’s in specified position*/
temp = temp % 2 ? 1 : 0 ;
hchar_a[tmpoffset] |= (temp & 1) << (7-tmpcurbitNo);
temp = 0;
}
/* for(i=0; i<= (totalbits/8) ; i++){
printf(„%x\n“,hchar_a[i]);
}*/
/*format and print in mathematical polynomial form*/
curbitNo = 0;
offset = 0;
for(i=totalbits; i>=1 ; i–){
if(hchar_a[offset]>>(7-curbitNo) & 1){
if(setFlag)
printf(„+“);
if(i-1)
printf(„x^(%d)“,i-1);
else
printf(„1“);
setFlag = 1;
}
curbitNo++;
if(curbitNo == 8){
curbitNo = 0;
offset++;
}
}
printf(„\n“);
}
/**
* FUNCTION NAME : isPowerOfTwo
*
* FILENAME : HammingCodeGen_v1.1.c
*
* @param : num to check
*
* @brief : to check if num is power of two
*
* @return : int
*/
int isPowerOfTwo(int n)
{
if (n == 0)
return 0;
while (n != 1)
{
if (n%2 != 0)
return 0;
n = n/2;
}
return 1;
}
Neueste Kommentare